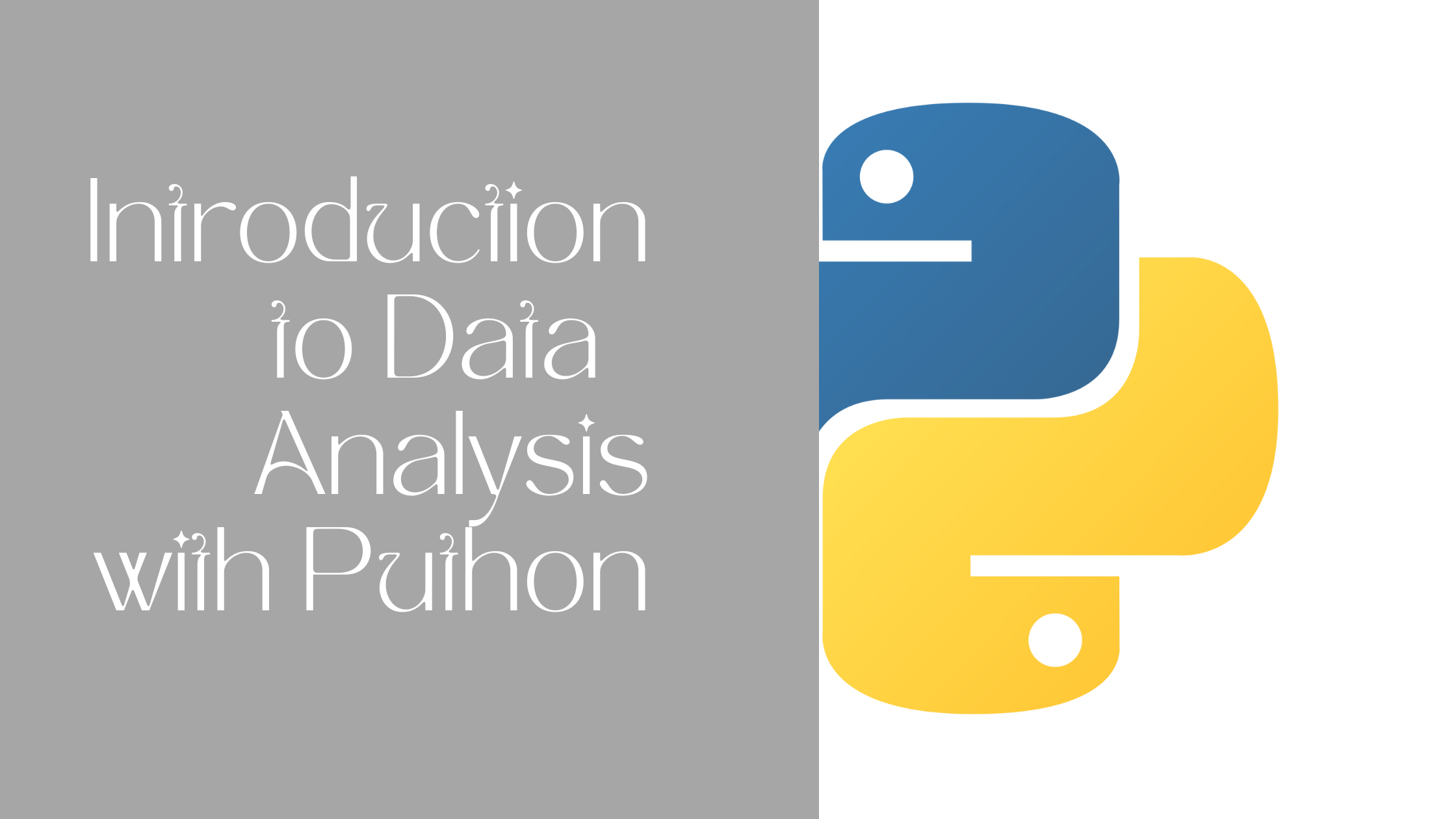
Introduction to Data Analysis with Python
In today’s data-driven world, the ability to extract meaningful insights from data is a valuable skill. Python, a versatile and widely-used programming language, has become a popular choice for data analysis. This blog post serves as an introductory guide to data analysis with Python, shedding light on its significance and fundamental concepts.
Why Python for Data Analysis?
Python’s popularity in the data analysis community can be attributed to several factors:
Open Source: Python is open source and free to use. This lowers the entry barrier for aspiring data analysts and data scientists.
Rich Ecosystem: Python boasts a rich ecosystem of libraries and tools, such as NumPy, Pandas, Matplotlib, and Jupyter, which are essential for data analysis tasks.
Community Support: A large and active community of Python users contributes to its continuous development and provides a wealth of resources and tutorials.
Cross-Platform Compatibility: Python runs on multiple operating systems, making it accessible to a broad audience.
Key Concepts in Data Analysis:
Before delving into Python’s data analysis capabilities, it’s crucial to understand some key concepts:
- Data Cleaning: The process of preparing data for analysis, which includes handling missing values, outliers, and formatting issues.
- Exploratory Data Analysis (EDA): An initial step in data analysis where you explore and summarize the data to gain insights and detect patterns.
- Data Visualization: Using charts and graphs to represent data visually, making it easier to interpret and communicate findings.
- Statistical Analysis: Applying statistical methods to understand data, including measures of central tendency, dispersion, and hypothesis testing.
- Machine Learning: Leveraging algorithms to make predictions or classify data based on patterns and relationships.
Getting Started with Python for Data Analysis:
To start your journey into data analysis with Python, you’ll need to:
- Install Python: Download and install Python from the official website (https://www.python.org/).
- Install Data Analysis Libraries: Use Python’s package manager, pip, to install libraries like NumPy, Pandas, and Matplotlib.
- Explore Jupyter Notebooks: Jupyter notebooks provide an interactive environment for data analysis. You can install them using pip as well.
- Learn Python Fundamentals: Familiarize yourself with Python’s basic syntax, data types, and control structures.
Data Cleaning:
Data cleaning is an essential step in the data analysis process. It involves handling missing data, removing duplicates, and ensuring the data is in a format suitable for analysis.
Code Sample: Handling Missing Data with Pandas
import pandas as pd
# Create a sample DataFrame with missing data
data = {‘A’: [1, 2, None, 4, 5],
‘B’: [None, 2, 3, 4, 5]}
df = pd.DataFrame(data)
# Check for missing values
print(df.isnull())
# Drop rows with missing values
df.dropna(inplace=True)
# Fill missing values with a specific value
df.fillna(0, inplace=True)
Exploratory Data Analysis (EDA):
EDA involves understanding the data by visualizing and summarizing it. It helps you identify patterns, outliers, and gain initial insights.
Code Sample: Basic EDA with Pandas and Matplotlib
import pandas as pd
import matplotlib.pyplot as plt
# Load your dataset
df = pd.read_csv(‘your_data.csv’)
# Summary statistics
print(df.describe())
# Histogram for a numerical variable
plt.hist(df[‘Age’], bins=20)
plt.xlabel(‘Age’)
plt.ylabel(‘Frequency’)
plt.title(‘Age Distribution’)
plt.show()
# Boxplot for numerical variables
df.boxplot(column=[‘Age’, ‘Income’])
plt.title(‘Boxplot of Age and Income’)
plt.show()
Data Visualization:
Data visualization is crucial for conveying insights effectively. Matplotlib and Seaborn are popular libraries for creating various types of charts and plots.
Code Sample: Creating a Scatter Plot with Matplotlib
import matplotlib.pyplot as plt
# Sample data
x = [1, 2, 3, 4, 5]
y = [10, 15, 13, 18, 22]
# Create a scatter plot
plt.scatter(x, y, label=’Data Points’, color=’blue’, marker=’o’)
plt.xlabel(‘X-axis’)
plt.ylabel(‘Y-axis’)
plt.title(‘Scatter Plot Example’)
plt.legend()
plt.show()
Code Sample: Creating a Bar Chart with Matplotlib
import matplotlib.pyplot as plt
# Sample data
categories = [‘Category A’, ‘Category B’, ‘Category C’]
values = [30, 45, 60]
# Create a bar chart
plt.bar(categories, values, color=’green’)
plt.xlabel(‘Categories’)
plt.ylabel(‘Values’)
plt.title ‘Bar Chart Example’
plt.show()
These code samples provide a comprehensive overview of data analysis with Python, covering data cleaning, exploratory data analysis, and data visualization. As you continue your data analysis journey, you’ll explore more advanced techniques and libraries to handle complex data analysis tasks. Stay tuned for more insights and practical tips as we delve deeper into Python’s data analysis capabilities in future blog posts!